Solved: Magick++ Version of 3 image masked alpha composition
Solved: Magick++ Version of 3 image masked alpha composition
Hi everybody,
currently I am trying to figure out how to write a c++ program using the magick++ libs to recreate the behaviour for the "special three image form of Masked Alpha Composition" mentioned in http://www.imagemagick.org/Usage/channe ... ed_compose.
As far as I understood, parts of the idea is by using the mask image with both the Multiply composite flag (like in image1.composite( image2, placement, MultiplyCompositeOp );) and the Screen composite flag.
Although I found the MultiplyCompositeOp define for magick++, I couldn't find the 'convert -composite Screen' equivalent.
Did I miss something here or is this method not available?
Has anyone a piece of c++ code that actually does the same like the 3 image form from above?
Any help or hint would be greatly appreciated.
Olli
currently I am trying to figure out how to write a c++ program using the magick++ libs to recreate the behaviour for the "special three image form of Masked Alpha Composition" mentioned in http://www.imagemagick.org/Usage/channe ... ed_compose.
As far as I understood, parts of the idea is by using the mask image with both the Multiply composite flag (like in image1.composite( image2, placement, MultiplyCompositeOp );) and the Screen composite flag.
Although I found the MultiplyCompositeOp define for magick++, I couldn't find the 'convert -composite Screen' equivalent.
Did I miss something here or is this method not available?
Has anyone a piece of c++ code that actually does the same like the 3 image form from above?
Any help or hint would be greatly appreciated.
Olli
Last edited by The_Chaos on 2010-08-30T00:59:45-07:00, edited 3 times in total.
-
- Posts: 1015
- Joined: 2005-03-21T21:16:57-07:00
Re: Magick++ Version of 3 image masked alpha composition
I have a MagickWand example in C of how to do that but it involves resorting to MagickCore to set an image mask.
http://members.shaw.ca/el.supremo/Magic ... ipmask.htm
Pete
http://members.shaw.ca/el.supremo/Magic ... ipmask.htm
Pete
Sorry, my ISP shutdown all personal webspace so my MagickWand Examples in C is offline.
See my message in this topic for a link to a zip of all the files.
See my message in this topic for a link to a zip of all the files.
Re: Magick++ Version of 3 image masked alpha composition
Hi el_supremo,
thank you very much for your help and hints! This is exactly what I needed!
Just for future references and in case somebody wants or needs the same thing, here is my piece of code that works:
Once again, thank you very much for your help!
Greetings,
Olli
thank you very much for your help and hints! This is exactly what I needed!

Just for future references and in case somebody wants or needs the same thing, here is my piece of code that works:
Code: Select all
#include <Magick++.h>
#include <iostream>
using namespace std;
using namespace Magick;
int main(int argc,char **argv)
{
// create image objects for use
Image src, dest, mask;
try {
// read image files into objects
dest.read( "imageWithPlaceToRenderPhotoIn.png" );
mask.read( "imageWithMaskForPhotoPlace.png" );
src.read( "photoToRenderIn.jpg" );
// create parameters for image distortion of the src image
double distortParams[] = {0,0, 82.5,554.5, 0,1200, 532.5,764.5, 800,1200, 786.5,256.5, 800,0, 422.5,118.5};
// set surrounding pixels to transparent
src.virtualPixelMethod(TransparentVirtualPixelMethod);
// distort the src image to fit inside the masked area
src.distort(MagickCore::PerspectiveDistortion, 16, distortParams, 0);
// negate the mask, since we need black pixels where content has to be seen
// although the mask is made with white pixels where content shines through
mask.negate();
// combine the destination image and the mask at the origin
Geometry placement(0,0,0,0);
dest.clipMask(mask);
dest.composite( src, placement, OverCompositeOp);
// Write the destination image to a file
dest.write( "finalImageWithPhotoRenderedIn.png" );
}
catch( Exception &error_ )
{
cout << "Caught exception: " << error_.what() << endl;
return 1;
}
return 0;
}
Greetings,
Olli
-
- Posts: 1015
- Joined: 2005-03-21T21:16:57-07:00
Re: SOLVED: Magick++ Version of 3 image masked alpha composi
And thank you for posting your code. I hadn't realized that MagickSetImageClipMask would work in that example. I had been looking for something that set wand->images->mask (which is what the convert/mogrify code does) but it seems that using MagickSetImageClipMask to set wand->images->clip_mask will still produce the desired result. I have updated the clipmask example.
Pete
Pete
Sorry, my ISP shutdown all personal webspace so my MagickWand Examples in C is offline.
See my message in this topic for a link to a zip of all the files.
See my message in this topic for a link to a zip of all the files.
Re: SOLVED: Magick++ Version of 3 image masked alpha composi
Hi Pete,

But I still got another question regarding the command line tools and the magick++ equivalent:
When I try to put an Overlay over an image to create shadows/highlights, I can use the following command: which uses a grayscale overlay image and darkens the part in the source image, where the overlay is dark and lightens the source where the overlay is bright. It looks like every grayscale value >127 darkens and <127 lightens the image up.
Now I was trying to figure out, what the equivalent for the magick++ solution above is. But neither OverCompositeOp is the right one (only get the overlay image) nor PlusCompositeOp is correct, since it darkens only.
And reading through the Image composition methods in the documentation was not leading to success.
In case you need some sample images, just let me know.
Thank you very much again for all your help!
Olli
Well thats the least I could doel_supremo wrote:And thank you for posting your code.

But I still got another question regarding the command line tools and the magick++ equivalent:
When I try to put an Overlay over an image to create shadows/highlights, I can use the following command:
Code: Select all
composite image.png overlay.png -compose Overlay final.png
Now I was trying to figure out, what the equivalent for the magick++ solution above is. But neither OverCompositeOp is the right one (only get the overlay image) nor PlusCompositeOp is correct, since it darkens only.
And reading through the Image composition methods in the documentation was not leading to success.
In case you need some sample images, just let me know.
Thank you very much again for all your help!
Olli
-
- Posts: 1015
- Joined: 2005-03-21T21:16:57-07:00
Re: SOLVED: Magick++ Version of 3 image masked alpha composi
Code: Select all
composite image.png overlay.png -compose Overlay final.png
In MagickWand the composite function is called like this:
Code: Select all
// This does the src (overlay) over the dest (background)
MagickCompositeImage(dest,src,OverlayCompositeOp,0,0);
Pete
Sorry, my ISP shutdown all personal webspace so my MagickWand Examples in C is offline.
See my message in this topic for a link to a zip of all the files.
See my message in this topic for a link to a zip of all the files.
Re: Magick++ Version of 3 image masked alpha composi
Hi again Pete,
sorry It took so long to answer, but I had quite some things to work on.
I checked again with the order of the composite command, and it doesn't work for me.
so I attached the image files used and also the final files that come out of the command line version (final_cmd.png) and the c++ version (final_cpp.png).
The command line version which works for me as expected is:
and this one results in this image:
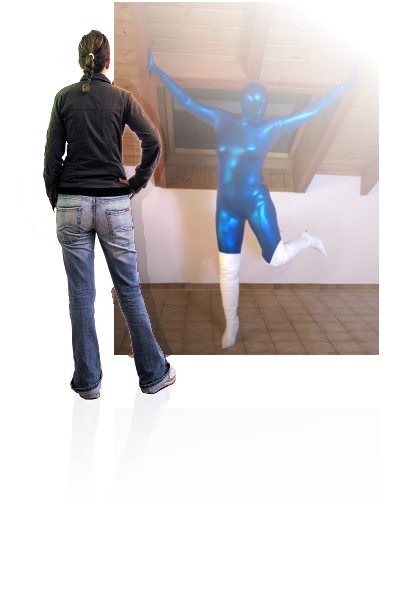
You can see the "background image" has lights and shadows at the persons side.
The C++ Code I used is:
which produces this image:

which doesn't contain the lights/shadows from the overlay image.
So I would love to have the c++ version to generate the same image with the overlay like final_cmd.png.
Maybe you find out what the problem is or probably it works better with the magicwand version.
You will find the complete file archive here.
I would appreciate any help on this thing
Greetings,
Olli
sorry It took so long to answer, but I had quite some things to work on.
I checked again with the order of the composite command, and it doesn't work for me.
so I attached the image files used and also the final files that come out of the command line version (final_cmd.png) and the c++ version (final_cpp.png).
The command line version which works for me as expected is:
Code: Select all
convert poster.png \( source.jpg -virtual-pixel transparent -distort Perspective '0,0 108,0 0,800 108,356 600,800 380,356 600,0 380,0' \) poster_mask.png -composite - | composite - poster_overlay5.png -compose Overlay final_cmd.png
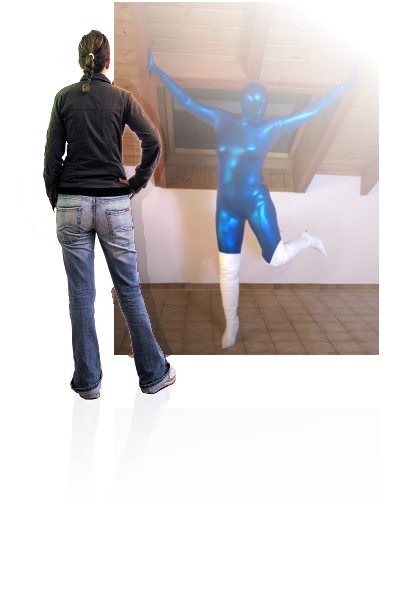
You can see the "background image" has lights and shadows at the persons side.
The C++ Code I used is:
Code: Select all
#include <Magick++.h>
#include <iostream>
using namespace std;
using namespace Magick;
int main(int argc,char **argv)
{
// create image objects for use
Image src, dest, mask, overlay;
try {
// define distorsion points
double distortParams[] = {0,0, 108,0, 0,800, 108,356, 600,800, 380,356, 600,0, 380,0};
// read image files into objects
dest.read( "poster.png" );
mask.read( "poster_mask.png" );
overlay.read( "poster_overlay5.png" );
src.read( "source.jpg" );
// set surrounding pixels to transparent
src.virtualPixelMethod(TransparentVirtualPixelMethod);
// distort the src image to fit inside the masked area
src.distort(MagickCore::PerspectiveDistortion, 16, distortParams, 0);
// negate the mask, since we need black pixels where content has to be seen
// although the mask is made with white pixels where content shines through
mask.negate();
// combine the destination image and the mask at the origin
Geometry placement(0,0,0,0);
dest.clipMask(mask);
dest.composite( src, placement, OverCompositeOp);
// Put the overlay on top of the image.
overlay.composite( dest, placement, OverCompositeOp);
// Write the destination image to a file
overlay.write( "final_cpp.png" );
}
catch( Exception &error_ )
{
cout << "Caught exception: " << error_.what() << endl;
return 1;
}
return 0;
}

which doesn't contain the lights/shadows from the overlay image.
So I would love to have the c++ version to generate the same image with the overlay like final_cmd.png.
Maybe you find out what the problem is or probably it works better with the magicwand version.
You will find the complete file archive here.
I would appreciate any help on this thing

Greetings,
Olli
-
- Posts: 1015
- Joined: 2005-03-21T21:16:57-07:00
Re: Magick++ Version of 3 image masked alpha composition
Hi Olli,
Your second composite should use OverlayCompositeOp.
Pete
Your second composite should use OverlayCompositeOp.
Pete
Sorry, my ISP shutdown all personal webspace so my MagickWand Examples in C is offline.
See my message in this topic for a link to a zip of all the files.
See my message in this topic for a link to a zip of all the files.
SOLVED: Re: Magick++ Version of 3 image masked alpha composi
Hi again Pete,
thank you very much for your fast help... that was really easy!
I somehow didn't find this one, don't know why.
Once again I really appreciate your help!
Greetings,
Olli
thank you very much for your fast help... that was really easy!
I somehow didn't find this one, don't know why.
Once again I really appreciate your help!
Greetings,
Olli